TaskFlowAI
TaskFlowAI is a lightweight, intuitive, and flexible framework for creating AI-driven task pipelines and multi-agent teams, centered around the concept of Tasks rather than conversation patterns.
Core Principles
TaskFlowAI is built around the concept of task completion, rather than conversation patterns. It has a modular architecture with interchangeable components. It's meant to be lightweight with minimal dependencies, and it offers transparency through a flat hierarchy and full prompt exposure.
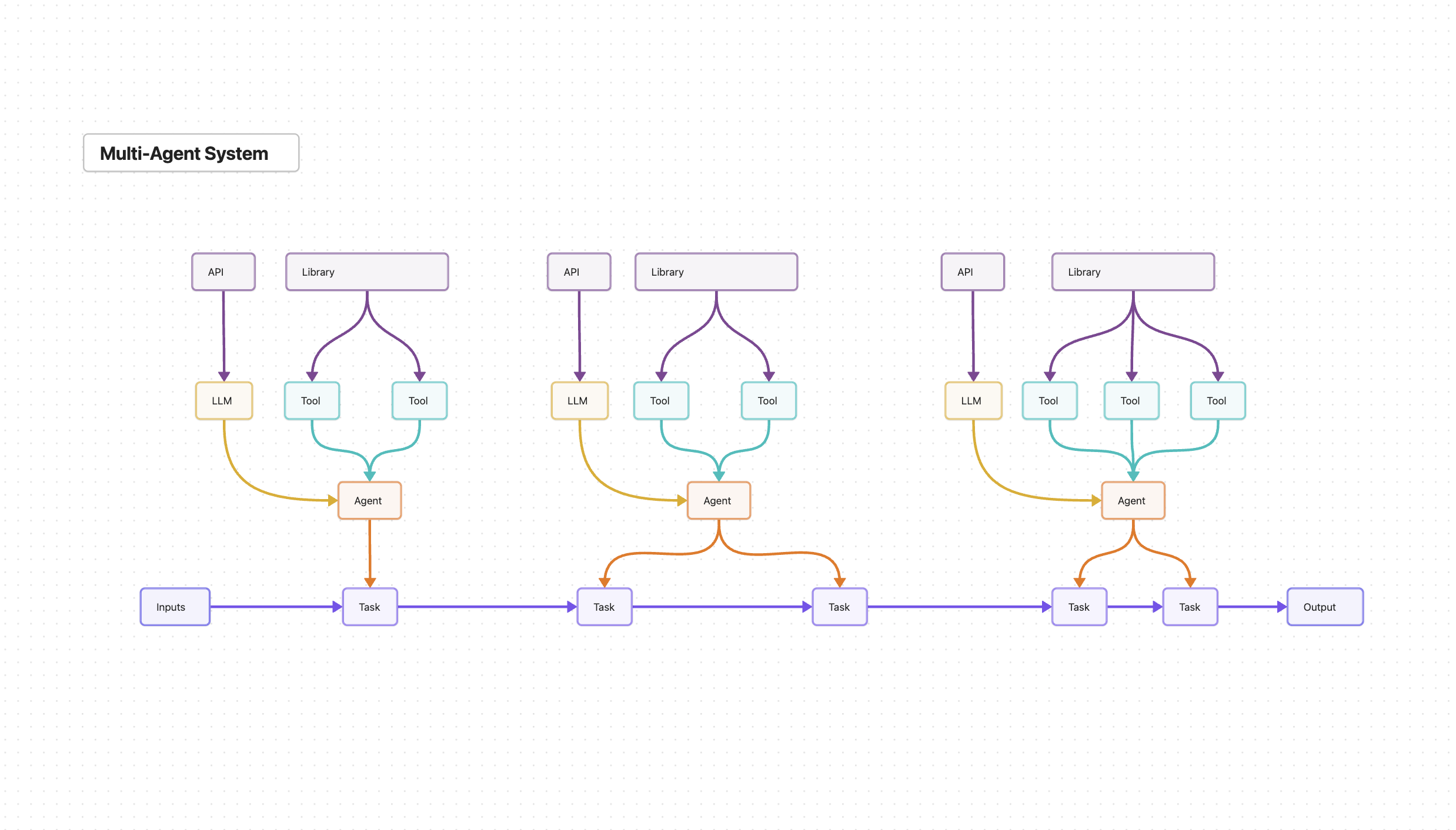
Core Components
Tasks
Tasks are the fundamental building blocks of TaskflowAI. Each task represents a single, discrete unit of work to be performed by a Large Language Model. They include an optional context field for providing relevant background information, and an instruction that defines the core purpose of the task.
Agents
An Agent in Taskflowai represents a specific role or persona with a clear goal. It can have optional attributes, and is powered by a selected language model (LLM). This structure allows Agents to maintain a consistent persona across multiple tasks. Agents can also be assigned tools, which are specific deterministic functions that the agent can use to interact with libraries, APIs, the internet, and more.
Tools
Tools in TaskFlowAI are wrappers around external services or APIs, as well as utilities for common operations. You can link tools together with tasks to create structured, deterministic AI-integrated pipelines, offering precise control over the AI's actions in scenarios that require predictable workflows. Or, you can directly assign tools to agents, and the agents to tasks, enabling more autonomous, self-determined tool use. In this mode, AI Agents can independently choose and utilize tools to complete their assigned tasks.
Language Models
TaskFlowAI supports various Language Models out-of-the-box, including models from OpenAI, Anthropic, open source models from OpenRouter, Groq, and Ollama where they can be locally hosted on device.
Getting Started
To begin using TaskFlowAI:
- Create a folder for your TaskflowAI projects
- Create a .env file with your relevant API Keys
- In your new folder, set up a virtual environment and install taskflowai
python -m venv venv
source venv/bin/activate # On Windows use: venv\Scripts\activate
pip install taskflowai
Once you have installed TaskflowAI, you can start building your task flows.
from taskflowai import Task, Agent, WebTools, OpenRouterModels
researcher = Agent(
role="research assistant",
goal="answer user queries",
attributes="thorough in web research",
tools={WebTools.serper_search},
llm=OpenRouterModels.haiku
)
def research_task(agent, topic):
return Task.create(
agent=agent,
instruction=f"Research {topic} and provide a summary of the top 3 results."
)
def main():
topic = input("Enter a topic to research: ")
response = research_task(researcher, topic)
print(response)
if __name__ == "__main__":
main()
Multi-Agent Teams
TaskFlowAI enables the creation of powerful multi-agent teams by assigning tasks to agents equipped with specific tools. This approach facilitates complex workflows and collaborative problem-solving, allowing you to tackle intricate challenges that require diverse skills and knowledge.
In a multi-agent team, each agent is designed with a specialized role, a set of tools, and specific expertise. By combining these agents, you can create AI workflows capable of handling a wide range of tasks, from research and analysis to problem-solving and code generation.
Streamlit UI Kit
TaskFlowAI UI is a powerful set of user interface components designed to work seamlessly with the TaskFlowAI framework. It provides developers with easy-to-use tools for creating interactive and engaging interfaces for AI-powered applications. It is built with Streamlit and includes two components: ChatUI and FormUI. ChatUI is a chat-based interface component that enables multi-message conversations with TaskFlowAI agents. FormUI is a form-based workflow component that allows developers to create structured, multi-step processes.
The UI wrappers can be installed with pip:
pip install taskflowai-ui
ChatUI
A chat-based interface component that enables multi-message conversations with TaskFlowAI agents.
ChatUI offers:
- Real-time interaction with AI agents
- Automatic rendering of messages and tool calls
- Persistent conversation history
FormUI
A form-based workflow component that allows developers to create structured, multi-step processes.
FormUI provides:
- Automatic form generation based on defined input fields
- Sequential execution of workflow steps
- Visualization of results and tool calls for each step
These components simplify the process of building user-friendly interfaces for TaskFlowAI applications, allowing developers to focus on creating powerful AI workflows while TaskFlowAI UI handles the presentation layer.
Whether you're building a conversational AI assistant or a complex, multi-step AI workflow, TaskFlowAI UI offers the tools you need to create polished, professional interfaces with minimal effort.